How Itura Works
Deploy your agentic application to a secure, serverless endpoint in 3 easy steps. Focus on your code, let Itura handle the rest.
From Code to Cloud in Minutes
Prepare Your Code
Add a Flask /run
endpoint to invoke your agent and a requirements.txt
file for dependencies. Itura uses these to build and run your agent.
from flask import Flask, request, jsonify
import os
app = Flask(__name__)
@app.route("/run", methods=["POST"])
def run_agent_endpoint():
data = request.get_json() # Pass parameters to the agent
env_vars = os.environ # Access environment variables
agent_output = my_agent_logic(data, env_vars)
return jsonify(agent_output)
def my_agent_logic(params, env_vars):
# Print statements are automatically capture by Itura
print(f"{len(params)} parameters and {len(env_vars)} env variables received")
# TODO: Implement your agent logic here using your favorite stack
return {"message": "Agent executed successfully!", "output": "Agent output"}
Connect Your GitHub Repo
Link the GitHub repository containing your agent code (including the Flask app and requirements.txt
). Itura automatically accesses the code, packages it into a container, and deploys it.
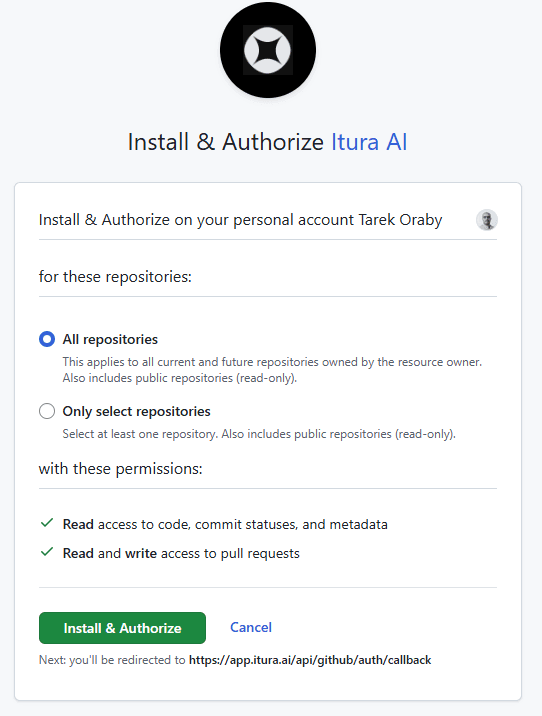
Add Environment Variables
Securely configure secrets (like API keys or configuration settings) your agent needs at runtime via the Itura dashboard. These are injected securely when your agent runs.

Deployment Complete! 🎉
That's it! Itura handles the rest. Your agent is automatically deployed to a unique, secure URL (like https://your-agent.agent.itura.ai
) with an API key. You can monitor execution history, logs, and status directly in the Itura UI. New commits to your connected repository trigger automatic re-deployments.
curl -X POST https://your-agent-name.agent.itura.ai/run \
-H "Authorization: Bearer <your_api_key>" \
-H "Content-Type: application/json" \
-d '{"input": "Data for your agent"}'
Key Advantages
Flexible
Supports any Python framework, library, or LLM via the Flask `/run` endpoint.
Agent-Focused
Manages Python dependencies, secure environment variable injection, serverless execution suitable for agent tasks.
Serverless & Scalable
No server management; scales automatically based on workload.
Secure
Isolated containerized execution environment; encrypted communication.
Automatic Updates
Integrates with GitHub for continuous deployment on every commit.
Cost-Effective
Pay only for the compute time used by your agent invocations.
Technical Overview
Runtimes
Python 3.9, 3.10, 3.11, 3.12, 3.13.
Frameworks
Any Python framework/library via Flask `/run`.
Resources
1GB RAM, 10 GB temporary storage per invocation.
Max Execution Time
10 minutes per invocation.
Code Size Limit
1 GB per agent (repository size).
Logging
Captured stdout/stderr; viewable in the Itura UI.